[RGSS3] [SOLVED] YANFLY COMMAND EQUIP & LUNA ENGINE WORKS
Posts
Red_Nova
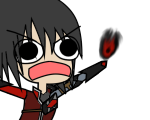
Sir Redd of Novus: He who made Prayer of the Faithless that one time, and that was pretty dang rad! :D
9192
author=GreatRedSpirit
The quickest and dirtiest way would be to just move the window offscreen and inactive (iirc it's @status_window.active = false ?) so it doesn't respond to input. I don't remember how window cleanup works in Ace atm though and I can't check it 'till I get home from work at the end of the day.
Unfortunately, that still follows the issue of the window not being set to false until after Scene_Equip is over.
Red_Nova
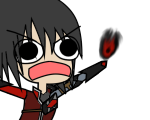
Sir Redd of Novus: He who made Prayer of the Faithless that one time, and that was pretty dang rad! :D
9192
Sure thing.
class Object def deep_clone return @deep_cloning_obj if @deep_cloning @deep_cloning_obj = clone @deep_cloning_obj.instance_variables.each do |var| val = @deep_cloning_obj.instance_variable_get(var) begin @deep_cloning = true if(val.is_a? Class) val = val else val = val.deep_clone end rescue TypeError next ensure @deep_cloning = false end @deep_cloning_obj.instance_variable_set(var, val) end deep_cloning_obj = @deep_cloning_obj @deep_cloning_obj = nil return deep_cloning_obj end end class Array def deep_clone return map { |x| x.deep_clone } end end class Numeric def deep_clone return self end end class FalseClass def deep_clone return self end end class TrueClass def deep_clone return self end end class NilClass def deep_clone return self end end class Window_EquipItem < Window_ItemList #-------------------------------------------------------------------------- # overwrite method: update_help #-------------------------------------------------------------------------- def update_help super return if @actor.nil? return if @status_window.nil? return if @last_item == item @last_item = item temp_actor = @actor.deep_clone temp_actor.force_change_equip(@slot_id, item) @status_window.set_temp_actor(temp_actor) end end class Scene_Battle < Scene_Base #-------------------------------------------------------------------------- # overwrite method: command_equip #-------------------------------------------------------------------------- def command_equip remove = [] fixed_slot = YEA::COMMAND_EQUIP::EQUIP_FIXEDSLOT Graphics.freeze @info_viewport.visible = false hide_extra_gauges if $imported["YEA-BattleEngine"] @actor_command_window.visible = false SceneManager.snapshot_for_background actor = $game_party.battle_members[@status_window.index] for etype_id in fixed_slot if not actor.equip_type_fixed?(etype_id) actor.actor.fixed_equip_type.push(etype_id) remove.push(etype_id) end end $game_party.menu_actor = actor previous_equips = actor.equips.clone index = @actor_command_window.index oy = @actor_command_window.oy #--- SceneManager.call(Scene_Equip) SceneManager.scene.main SceneManager.force_recall(self) #--- show_extra_gauges if $imported["YEA-BattleEngine"] actor.set_equip_cooldown if previous_equips != actor.equips @info_viewport.visible = true @actor_command_window.visible = true @status_window.refresh @actor_command_window.setup(actor) @actor_command_window.select(index) @actor_command_window.oy = oy for etype_id in remove actor.actor.fixed_equip_type.delete(etype_id) end perform_transition next_command if YEA::COMMAND_EQUIP::EQUIP_SKIPTURN end alias nova_command_equip command_equip def command_equip hide_battler_picture nova_command_equip show_battler_picture @ftb_gauge.show if $imported["YEA-BattleSystem-FTB"] end end # Scene_Battle #-------------------------------------------------------------------------- # * new method: show_battler_picture #-------------------------------------------------------------------------- def show_battler_picture @leaf = Sprite.new @leaf.bitmap = Cache.picture("ActorSelection_" + BattleManager.actor.class_id.to_s) @leaf.y = 90 @status_window.open end #-------------------------------------------------------------------------- # * new method: hide_battler_picture #-------------------------------------------------------------------------- def hide_battler_picture @leaf.bitmap.dispose @leaf.dispose @status_window.close end
If it's just a Scene_Equip call then look into how it closes itself up since the @status_window in question is part of it. Since Luna breaks the default one I'd assume it replaces large swaths of it with its own stuff but there should still be a process where it cleans up its windows (although why some stuff is sticking around is weird, it should be collected by the GC eventually).
Can you PM me a version of your game where I can easily see and test the bug itself? I think I'm missing something and seeing it in action and its script will help me with a solution.
Can you PM me a version of your game where I can easily see and test the bug itself? I think I'm missing something and seeing it in action and its script will help me with a solution.
Red_Nova
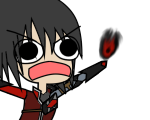
Sir Redd of Novus: He who made Prayer of the Faithless that one time, and that was pretty dang rad! :D
9192
Through PMs, we (and I really mean GRS) got the issue solved! Now there's no crashing and the battle HUD disappears when it's supposed to. Thanks, GRS!
EDIT: Once I do some more testing, I'll try to convert it so that it'll work with the base Luna Engine so other people can make use of this fix, too. There's a few modifications to work with just my game, you see.
EDIT: Once I do some more testing, I'll try to convert it so that it'll work with the base Luna Engine so other people can make use of this fix, too. There's a few modifications to work with just my game, you see.
Red_Nova
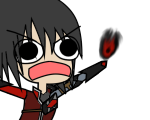
Sir Redd of Novus: He who made Prayer of the Faithless that one time, and that was pretty dang rad! :D
9192
Okay everyone, here's the patch for the Command Equip compatibility with all the modifications for my specific game taken out. Make sure to put this BELOW the command equip script. I tested this in a fresh copy of Luna Base version 1.03. If something causes the game to crash, let me know and we'll figure it out.
GreatRedSpirit pretty much scrapped most of my freshman code to get this to work, so credit him if you use this in your game (me too if you wanna be nice, but make sure GRS is on the list at least).
GreatRedSpirit pretty much scrapped most of my freshman code to get this to work, so credit him if you use this in your game (me too if you wanna be nice, but make sure GRS is on the list at least).
class Object def deep_clone return @deep_cloning_obj if @deep_cloning @deep_cloning_obj = clone @deep_cloning_obj.instance_variables.each do |var| val = @deep_cloning_obj.instance_variable_get(var) begin @deep_cloning = true if(val.is_a? Class) val = val else val = val.deep_clone end rescue TypeError next ensure @deep_cloning = false end @deep_cloning_obj.instance_variable_set(var, val) end deep_cloning_obj = @deep_cloning_obj @deep_cloning_obj = nil return deep_cloning_obj end end class Array def deep_clone return map { |x| x.deep_clone } end end class Numeric def deep_clone return self end end class FalseClass def deep_clone return self end end class TrueClass def deep_clone return self end end class NilClass def deep_clone return self end end class Window_EquipItem < Window_ItemList #-------------------------------------------------------------------------- # overwrite method: update_help #-------------------------------------------------------------------------- def update_help super return if @actor.nil? return if @status_window.nil? return if @last_item == item @last_item = item temp_actor = @actor.deep_clone temp_actor.force_change_equip(@slot_id, item) @status_window.set_temp_actor(temp_actor) end end class Scene_Battle < Scene_Base alias nova_command_equip command_equip def command_equip @actor_command_window.visible = false @status_window.close unless @status_window.nil? or @status_window.disposed? 6.times do update end # grs-get those fukkin windows to proc nova_command_equip @status_window.open @actor_command_window.visible = true @ftb_gauge.show if $imported["YEA-BattleSystem-FTB"] end end # Scene_Battle