[RMMV] ITEM ACQUISITION ANIMATION
Posts
Pages:
1
Hello again,
I made an animation for my game for when the character acquires something, sort of like in Zelda:
I'd like this animation to trigger every time the player acquires something. Of course, instead of an apple, I'd like the icon of the acquired item to show up on top of the character.
What would be the best way to go about doing this? If possible, please explain in details as I'm really a beginner with the engine.
Thank you!
I made an animation for my game for when the character acquires something, sort of like in Zelda:

I'd like this animation to trigger every time the player acquires something. Of course, instead of an apple, I'd like the icon of the acquired item to show up on top of the character.
What would be the best way to go about doing this? If possible, please explain in details as I'm really a beginner with the engine.
Thank you!
There's multiple ways, the way I'd do it is a battle animation with the item sprite going up in the air and having the charset actually animate along with it. Other methods would involve stuff like Show Picture commands but this method is serviceable enough.
First you'd want to do it in a common event (look in the database for the tab) so you can call it whenever you want using Flow Control: Common Event(page1) in regular events on the map.
For the first command in page2 Character: Show Animation... put the animation of the fruit going up in the air (might need to figure out how the battle animation editor works and how to put the fruit in a proper battle animation sheet with only one image). Make sure wait for completion is OFF, we'll want the character to do an animation at the same time.
Next part you'll want to do Movement: Set Movement Route (page2) on the player and then use Change Image to another character set. The art part of this is making the animation of the character putting their arms up in the air through various FACINGS for example (facing down is frame 1, facing right is frame 2, facing left is frame 3) so in the Set Movement Route list you'll want to use the turn down/left/right/up commands in the second column. If you want more than 4 frames you simply just switch to another "character". You'll probably want to put some wait commands inbetween the turns so it doesnt zip by instantly. You can also abuse the stepping animation to do simple animation loops for other stuff.
Something like this is what the result will look like:
Add a message and giving the item or whatever fluff you want. You may need to adjust the wait timings a bit to get the classic Zelda feel you're likely going for or add in sound effects or whatever. Also you'll probably have to switch the character's image back to normal after.
Now to make a different item appear each time will require an additional step. So with the default fruit animation simply copy paste the battle animation in a bunch of slots in the database. Then change the actual source image to something else for each one. This way you'll preserve the going up in the air animation. It does require making a separate image for each item however.
We're now going to need a variable that will hold an id of the item that you just got, we'll call it ItemGivenID. This is the variable that will decide which animation plays based on which item you want to give, and will also determine which item actually is given. So for example in a typical event like a treasure chest, you'll want to set the ItemGivenID variable to the item you want to give, we'll assume the fruit item is in slot 1 of the database. Then we'll put in the commonevent call for the thing we just did. So whenever you make an event that gives you an item, you'll have to set the id to the number you want to give and call the common event (easy to copy paste and edit that way).
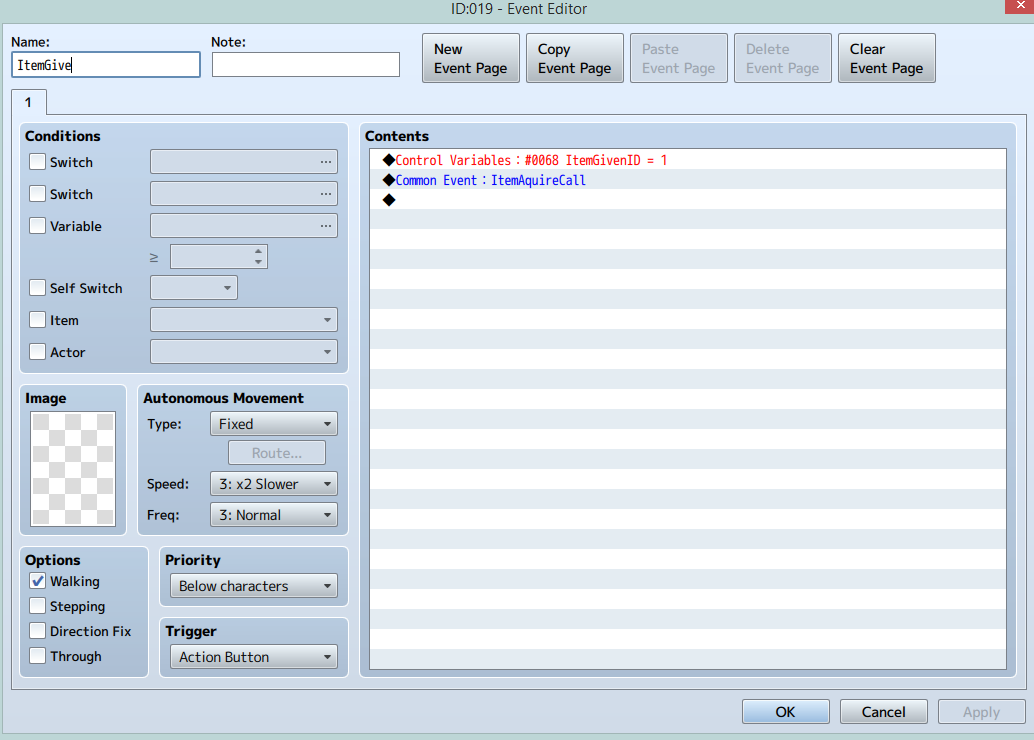
So going back to the common event we made before. We're going to want to change the animation, the message, and the actual item given all based on a single variable. So we're going to use Flow Control: Conditional Branch in page 1, and set it to ItemGivenID, and constant = 1, then copy paste the battle animation inside of it so it looks like this:

The character animation will be the same regardless of the item we picked up so we'll leave it alone. As for the message, we'll be doing the same thing we did with the battle animation. You can just copy paste the conditional branch you made before, delete the battle animation command and paste the message in there. As for giving the actual item to put in the inventory... well... MV doesn't have a command for giving you an item id based on a variable. So I'm going to drop a bit of scripting on you. Simply go to Advanced: Script... on page 3 and addthen change X to the ItemGivenID variable number (as in the number of the variable) and Y for the amount you wanna give (just put 1 for now). It should look something like this:

Now whenever you want to add a new item to the game, just copy paste the coneditional branches you made and change the values (right click on any command to see the hotkeys such as space to edit ctrl c to copy etc.). If you're having problems with the scripting command you can also add each item under each new conditional branch as well. It's just nice to do everything in one single command when you can.
So you'll have something like this:

It's a bit messy and there are ways to condense this a little better but if you're just starting out and figuring out the usefulness of conditional branches and common events this should be serviceable. Make sure to use Flow Control Comment(page1) if you want to put in notes to remember what you did.
Let me know if something doesn't make sense or if something doesnt work (I only tested this like once and didnt do any art for the example)
First you'd want to do it in a common event (look in the database for the tab) so you can call it whenever you want using Flow Control: Common Event(page1) in regular events on the map.
For the first command in page2 Character: Show Animation... put the animation of the fruit going up in the air (might need to figure out how the battle animation editor works and how to put the fruit in a proper battle animation sheet with only one image). Make sure wait for completion is OFF, we'll want the character to do an animation at the same time.
Next part you'll want to do Movement: Set Movement Route (page2) on the player and then use Change Image to another character set. The art part of this is making the animation of the character putting their arms up in the air through various FACINGS for example (facing down is frame 1, facing right is frame 2, facing left is frame 3) so in the Set Movement Route list you'll want to use the turn down/left/right/up commands in the second column. If you want more than 4 frames you simply just switch to another "character". You'll probably want to put some wait commands inbetween the turns so it doesnt zip by instantly. You can also abuse the stepping animation to do simple animation loops for other stuff.
Something like this is what the result will look like:
Add a message and giving the item or whatever fluff you want. You may need to adjust the wait timings a bit to get the classic Zelda feel you're likely going for or add in sound effects or whatever. Also you'll probably have to switch the character's image back to normal after.
Now to make a different item appear each time will require an additional step. So with the default fruit animation simply copy paste the battle animation in a bunch of slots in the database. Then change the actual source image to something else for each one. This way you'll preserve the going up in the air animation. It does require making a separate image for each item however.
We're now going to need a variable that will hold an id of the item that you just got, we'll call it ItemGivenID. This is the variable that will decide which animation plays based on which item you want to give, and will also determine which item actually is given. So for example in a typical event like a treasure chest, you'll want to set the ItemGivenID variable to the item you want to give, we'll assume the fruit item is in slot 1 of the database. Then we'll put in the commonevent call for the thing we just did. So whenever you make an event that gives you an item, you'll have to set the id to the number you want to give and call the common event (easy to copy paste and edit that way).
So going back to the common event we made before. We're going to want to change the animation, the message, and the actual item given all based on a single variable. So we're going to use Flow Control: Conditional Branch in page 1, and set it to ItemGivenID, and constant = 1, then copy paste the battle animation inside of it so it looks like this:
The character animation will be the same regardless of the item we picked up so we'll leave it alone. As for the message, we'll be doing the same thing we did with the battle animation. You can just copy paste the conditional branch you made before, delete the battle animation command and paste the message in there. As for giving the actual item to put in the inventory... well... MV doesn't have a command for giving you an item id based on a variable. So I'm going to drop a bit of scripting on you. Simply go to Advanced: Script... on page 3 and add
$gameParty.gainItem($dataItems[$gameVariables.value(X)], Y);
Now whenever you want to add a new item to the game, just copy paste the coneditional branches you made and change the values (right click on any command to see the hotkeys such as space to edit ctrl c to copy etc.). If you're having problems with the scripting command you can also add each item under each new conditional branch as well. It's just nice to do everything in one single command when you can.
So you'll have something like this:
It's a bit messy and there are ways to condense this a little better but if you're just starting out and figuring out the usefulness of conditional branches and common events this should be serviceable. Make sure to use Flow Control Comment(page1) if you want to put in notes to remember what you did.
Let me know if something doesn't make sense or if something doesnt work (I only tested this like once and didnt do any art for the example)
Thanks for the detailed answer.
If I don't want the character to face different directions and just use the face direction, then I can get rid of the West, East and North directions, right?
If I don't want the character to face different directions and just use the face direction, then I can get rid of the West, East and North directions, right?
If you only want one frame of animation then yes you would only need one facing. But the reason why I'm doing different facings is to not really make the character face in different directions per say, but just use it as frames of animation if that makes sense. You could just swap different parts of the charset, but it would be a waste of space if you left the 3 other rows of sprites empty.
Here's an example (it's for rpgmaker2003 but the principle is the same)
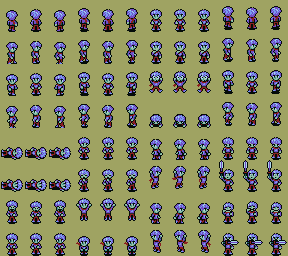
Here's an example (it's for rpgmaker2003 but the principle is the same)
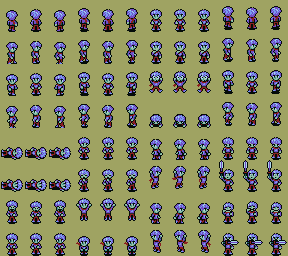
I'll be honest, I'm confused. I'm still very new to RPG Maker so that might be it.
I've done like you said and went to create the common event. Here's my question though:
When I'm making my animation, do I use the same animation template as I would for battle animations? From what I understand, each frame for animations is 192px/192px. Where do I put my character? On the bottom left corner of each square? Or am I using the wrong animation template? Am I supposed to use a character walking animation sheet? I think that's what I'm supposed to do but I'm not sure.
I've done like you said and went to create the common event. Here's my question though:
When I'm making my animation, do I use the same animation template as I would for battle animations? From what I understand, each frame for animations is 192px/192px. Where do I put my character? On the bottom left corner of each square? Or am I using the wrong animation template? Am I supposed to use a character walking animation sheet? I think that's what I'm supposed to do but I'm not sure.
The character walking animation sheet (known as img/characters in resources) is the template you'd want to work with for the actual character animations. The Battle Animation is just for the item itself to appear over the character. So when I say animation I meant in a general sense unless I'm referring to Battle Animation in particular.
Ah ok, thanks for clarifying.
But if I use the walking animation, won't I be limited to 4 frames for the animation (like for a walking animation). Because my animation is longer than that...
But if I use the walking animation, won't I be limited to 4 frames for the animation (like for a walking animation). Because my animation is longer than that...
With the Change Image in Set Movement Route you can switch over to another character slot and cycle the facing from there. Say you have an 8 frame animation you want to do, you can just use another set over and Turn down, turn left, turn right, turn up over and over.

edit: To be clear you're not using the "stepping" frames in the walking sheet, you're just using the static standing frames for poses. It's a bit unothordox but it's the way to change the characters sprite without any plugins needed.

edit: To be clear you're not using the "stepping" frames in the walking sheet, you're just using the static standing frames for poses. It's a bit unothordox but it's the way to change the characters sprite without any plugins needed.
Ahhh ok, I think I understand, you're sort of circumventing RPG Maker's limitation.

So out of curiosity since I'm on the topic.
You'll notice I've used the same frame twice on the sprite sheet. That's because I want this frame to last longer in the actual animation. I'm guessing RPG Make MV doesn't provide control over which frame lasts long in each animation so hopefully it'll come across ok. I think I'm on the right path at the moment, thanks for the patience. Hopefully that double-frame won't look weird or that quickframe with the eye blur.
I don't understand that part however.

So out of curiosity since I'm on the topic.
You'll notice I've used the same frame twice on the sprite sheet. That's because I want this frame to last longer in the actual animation. I'm guessing RPG Make MV doesn't provide control over which frame lasts long in each animation so hopefully it'll come across ok. I think I'm on the right path at the moment, thanks for the patience. Hopefully that double-frame won't look weird or that quickframe with the eye blur.
For the first command in page2 Character: Show Animation... put the animation of the fruit going up in the air (might need to figure out how the battle animation editor works and how to put the fruit in a proper battle animation sheet with only one image). Make sure wait for completion is OFF, we'll want the character to do an animation at the same time.
I don't understand that part however.
Actually you can decide how long each "facing" lasts with the Wait command in the Move Route and can set the amount of frames (5 frames being an example in my tutorial).

Alright, I've managed the first step at last.
As I said, it's just about figuring out how to have the item appear now. I don't know where and how the apple will appear but I'll give it a shot as a battle animation.
The problem with the battle animation is that it's super zoomed in which might turn out to be problematic.
Could I use another way than the battle animation? I find it's a super hassle to move every animation just right and I can't imagine messing with that with every single item. I managed the pick up animation, it works great.
You could use Show Picture / Move Picture for the item animation. You kind of have to fiddle with the coordinates as to where the picture will end up but since it's just an image translating up in the air it should do the trick. Should be somewhere on page 2 of event commands.
If you want show picture to be exactly where the player is you'll have to make two variables one called ItemX and one called ItemY (they dont have to be named a certain way btw, just for remembering what they do. A better personal name is DisplayItem in hindsight).
Set the X variable to Game Date > Screen X of Player, do the same for Y.
(Make sure Origin is Center)
Then in the Show Picture command, instead of inputting a direct designation just plug in the X and Y variables. This way the Picture wherever the hero is in relation to the screen.
However the picture will be right ON TOP of the hero so you'll have to subtract a number of pixels you want it to be above the player (X and Y coordinates follow a weird programming thing where negative Y is actually UP on the screen)
Then you'll want to move the picture, so after the Show Picture command subtract the amount of pixels you want it to move upwards. Then do a Move Picture command and input the same stuff as the Show Picture, but set the frames you want it to take to get there (each frame is 1/60th of a second)
(-40 correct the positions, -60 moves it up, +10 moves it down to give a settle)
Isolated, the result should look something like this. I added the additional move picture to make the animation less crude but there is a method to making the animation more exact pixel by pixel if you want to do crazy easing. Don't forget to erase picture at some point. Also if you want to variate the item image, you only need to do conditional branches on the show picture command inside the common event. The Move Picture commands don't care what you have for an image.
The main reason why I suggested battle animations earlier is that it didn't involve you having to set coordinates through variables to place it on the player. But this method I admit does not involve coming to grips with an editor and is at least more direct.
Set the X variable to Game Date > Screen X of Player, do the same for Y.
(Make sure Origin is Center)
Then in the Show Picture command, instead of inputting a direct designation just plug in the X and Y variables. This way the Picture wherever the hero is in relation to the screen.
However the picture will be right ON TOP of the hero so you'll have to subtract a number of pixels you want it to be above the player (X and Y coordinates follow a weird programming thing where negative Y is actually UP on the screen)
Then you'll want to move the picture, so after the Show Picture command subtract the amount of pixels you want it to move upwards. Then do a Move Picture command and input the same stuff as the Show Picture, but set the frames you want it to take to get there (each frame is 1/60th of a second)

Isolated, the result should look something like this. I added the additional move picture to make the animation less crude but there is a method to making the animation more exact pixel by pixel if you want to do crazy easing. Don't forget to erase picture at some point. Also if you want to variate the item image, you only need to do conditional branches on the show picture command inside the common event. The Move Picture commands don't care what you have for an image.
The main reason why I suggested battle animations earlier is that it didn't involve you having to set coordinates through variables to place it on the player. But this method I admit does not involve coming to grips with an editor and is at least more direct.
Pages:
1