COELOCANTH'S PROFILE
coelocanth
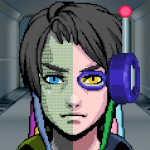
5448
Search
Filter
[RMMV] Need help with making the Level 5 Death Skill from FF5
Because you're using Yanfly plugins, it's actually easier.
Use YEP_SkillCore plugin and put this in your skill's note box:
For the damage formula itself, I'd suggest this:
but you could also just leave it blank if you prefer.
A skill that does no damage may show a miss or "there was no effect on goblin"
Another consideration is that the "addState" function is unconditional - your level 5 death spell will kill people even if they have death resist accessories equipped.
To account for resistances, you should instead use "itemEffectAddState", like this:
In this case it's a bit more complicated as you're building the data structure which would be in the database entry for the skill in code.
And finally for people with more of a coder mindset, I think an elegant way would be to make custom hit chances on skills.
This function in Game_Action could be overridden:
And since it has both the target as a an argument and the user as this.subject(), a custom hit chance could be written that takes levels into account.
Then the skill in the database could just have "add state: death 100%", but a custom hit chance in the notes like this:
In that case, the skill would miss anyone whose level isn't divisible by 5, and hit if it is (with the death state being added only to targets that were hit)
Writing the plugin for that is left as an exercise for the reader.
(post edited to correct typos in code)
Use YEP_SkillCore plugin and put this in your skill's note box:
<Post-Damage Eval>
if(b.level % 5 == 0) {
b.addState(1);
}
</Post-Damage Eval>
(b.level % 5 == 0) ? 9999 : 0
A skill that does no damage may show a miss or "there was no effect on goblin"
Another consideration is that the "addState" function is unconditional - your level 5 death spell will kill people even if they have death resist accessories equipped.
To account for resistances, you should instead use "itemEffectAddState", like this:
<Post-Damage Eval>
if(b.level % 5 == 0) {
this.itemEffectAddState(target, {
code: Game_Action.EFFECT_ADD_STATE,
dataId: 1, // dataId is the state number (0 for "normal attack" to use weapon states)
value1: 1, // value1 is the normalized chance to apply the state (1 = 100%)
value2: 0 // value2 is not used for states
});
}
</Post-Damage Eval>
In this case it's a bit more complicated as you're building the data structure which would be in the database entry for the skill in code.
And finally for people with more of a coder mindset, I think an elegant way would be to make custom hit chances on skills.
This function in Game_Action could be overridden:
Game_Action.prototype.itemHit = function(target) {
if (this.isPhysical()) {
return this.item().successRate * 0.01 * this.subject().hit;
} else {
return this.item().successRate * 0.01;
}
};
And since it has both the target as a an argument and the user as this.subject(), a custom hit chance could be written that takes levels into account.
Then the skill in the database could just have "add state: death 100%", but a custom hit chance in the notes like this:
<custom success rate>
b.level % 5 == 0 ? 1 : 0
</custom success rate>
Writing the plugin for that is left as an exercise for the reader.
(post edited to correct typos in code)
Font Texture
If you're using a large number of colors then the texture cache could grow excessively, But if you're using '#FFFFFF' every time then it should be using the cached texture for that color every time and generating it only once.
That's the variable _coloredFontTextures if you want to check it in the debugger.
Let me know if you find anything - the main drawing code is just a series of context.drawImage calls to copy rectangles of pixels from the font texture to the window texture. Nothing particularly clever.
That's the variable _coloredFontTextures if you want to check it in the debugger.
Let me know if you find anything - the main drawing code is just a series of context.drawImage calls to copy rectangles of pixels from the font texture to the window texture. Nothing particularly clever.
Isle of Dread Review
The graphics are indeed the XP RTP, with some conversion of the auto tiles. Except for the tree canopies, which are from REFMAP.
Swap in 3 Middle with Who?
Font Texture
author=SpellcraftQuill
https://i.postimg.cc/Qxz8qFQK/image.pngText gets cut off in a message box.
Uploaded a new version with fixes for this.
When updating an existing project, be sure to open the plugin settings and save them.
* The clipping box can be expanded by a configurable size in each direction. The default is 2 font pixels, which is usually enough to allow outlines not to be cut off.
* clipping can be turned off entirely if you prefer (which will allow text to overflow the max width requested).
Is there a way to skip the [monster] emerges message in RPG Maker MZ?
A further thought:
Because the game message system is used to display that text box, you can use text codes to auto dismiss the message.
e.g. in the "terms" section of the database, change the Emerge text to "%1 emerged!\.\^"
This would display the message, wait 0.25 second, then dismiss the message automatically.
If you want to skip the messages entirely, do what Marrend said and override either BattleManager.displayStartMessages or BattleManager.startBattle.
Because the game message system is used to display that text box, you can use text codes to auto dismiss the message.
e.g. in the "terms" section of the database, change the Emerge text to "%1 emerged!\.\^"
This would display the message, wait 0.25 second, then dismiss the message automatically.
If you want to skip the messages entirely, do what Marrend said and override either BattleManager.displayStartMessages or BattleManager.startBattle.
RPG Maker MV - What's the easiest way to have an enemy have multiple actions per turn?
Go to the enemies traits, and add "action times+ 100%" for each extra action per turn you want them to have.
If the chance is less than 100%, it's random whether they get an extra action or not.
If the chance is less than 100%, it's random whether they get an extra action or not.
[RMMZ] Calling on a common event after a fight
You could have an auto run or parallel common event activated by a switch, and turn that switch on during the battle via a battle event - it will run when returning to the map in that case.
Otherwise plugin is the best route - $gameTemp.reserveCommonEvent(id) queues an event to run on return to the map.
(MZ is a queue, MV has only one reserved event that replaces any previous one, IIRC)
Otherwise plugin is the best route - $gameTemp.reserveCommonEvent(id) queues an event to run on return to the map.
(MZ is a queue, MV has only one reserved event that replaces any previous one, IIRC)
EXCEED THE MAXIMUM LIMIT OF 4 CHARACTERS ON A TEAM ? [RMMZ]
author=NoobMaker
There is a data bank that classifies all the commands that can be integrated into a script ? as:
*Window_BattleStatus. prototype. maxCols
*Game_Action.prototype.applyCritica
*Game_Party. prototype. maxBattleMembers
*...
The core scripts in your game folder are presented in a readable form - if you look in the rmmz_windows.js you can find all the functions that are part of the base engine windows, rmmz_objects.js has all of the Game_xxx classes for actors, party, item etc.
If you study those, with a general javascript reference to look up anything you don't understand, you'll be able to figure out what functions are available and what they do.
[SOLVED] [RMVX ACE] Game font is not appearing in the exported game
Check that the custom font is in the fonts subfolder of the exported game.
If you have not removed the requirement completely, then the default font VL-Gothic-Regular.ttf should also be in the fonts folder.
If you've made an RTP free game, then everything should be in the subfolders of your game folder that is needed to run it.
If you have not removed the requirement completely, then the default font VL-Gothic-Regular.ttf should also be in the fonts folder.
If you've made an RTP free game, then everything should be in the subfolders of your game folder that is needed to run it.